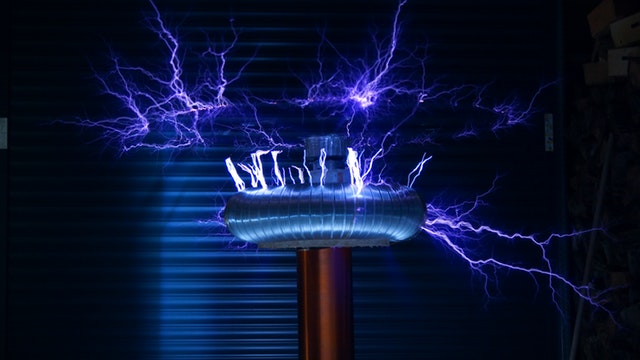
In my last PowerShell post, I hope I convinced you that PowerShell isn’t just for the server engineers. This time we’ll take a look at a few more cmdlets that can come in handy for network engineers.
A Ping of a Different Color
Ok so ping is not exactly revolutionary, but if you’re wanting to script a bunch of pings, PowerShell has you covered. In PowerShell, a ping is accomplished using the cmdlet Test-Connection. At its simplest form, it’s just like a ping command.
Test-Connection -ComputerName srv01
Of course, there’s no need to do that in PowerShell, but it can be useful for scripting. By adding the “-Quiet” parameter the results of Test-Connection becomes a simple boolean of true or false. This can then be used with if statements to only try to run an additional cmdlet if the Test-Connection succeeds.
Another way to use Test-Connection in scripting is to have it help you ping multiple computers. One way is to list multiple hosts after the “-ComputerName” parameter separated by commas. Programatically it may also make sense to do something like the following example.
For ($i=1; $i -le 255; $i++) {
Test-Connection -ComputerName 192.168.1.$i
}
That code will iterate through IP addresses pinging each, in turn, starting at 192.168.1.1 and ending with 192.168.1.254. The next step is to make the cmdlet ping a destination from multiple hosts.
Test-Connection -Source srv01, srv02, srv03 -ComputerName otherSrv01
More about the Test-Connection cmdlet and the various data forms that it can return can be found at Microsoft’s documentation site.
What’s Your Address?
Another common task is to retrieve and/or set the IP address for a network interface. PowerShell does not disappoint and provides the cmdlets for the job. The Get-NetIPConfiguration cmdlet will list the IP Address, interface, default gateway, and DNS servers.
Get-NetIPConfiguration
InterfaceAlias : Ethernet
InterfaceIndex : 1
InterfaceDescription : Intel(R) Ethernet Connection (2) I219-LM
NetProfile.Name : packitforwarding.com 2
IPv4Address : 192.0.2.2
IPv4DefaultGateway : 192.0.2.1
DNSServer : 208.67.222.222
208.67.220.220
You may have noticed that there was one thing missing that you may want to know about. What is the subnet mask? Well at least at the time I’m writing this, there is not a native PowerShell way to get the subnet mask, but you can get the prefix length. The Get-NetIPAddress cmdlet will get the prefix and it also will add information about the current remaining life of the DHCP lease as an added bonus.
Get-NetIPAddress
IPAddress : 192.0.2.2
InterfaceIndex : 1
InterfaceAlias : Ethernet
AddressFamily : IPv4
Type : Unicast
PrefixLength : 24
PrefixOrigin : Dhcp
SuffixOrigin : Dhcp
AddressState : Preferred
ValidLifetime : 5.17:21:00
PreferredLifetime : 5.17:21:00
SkipAsSource : False
PolicyStore : ActiveStore
There are two cmdlets for setting the IP address. The first one Set-NetIPAddress allows you to enable or disable DHCP. The second one New-NetIPAddress allows you to set a static IP address.
New-NetIPAddress -InterfaceIndex 1 -IPAddress 192.0.2.5 -PrefixLength 24 -DefaultGateway 192.0.2.1
Set-NetIPAddress -InterfaceIndex 1 -DHCP Enabled
While you will probably never have a need to use a lot of these cmdlets on a one-off situation, their power comes from the ability to use them as part of a larger script. In one case I was able to use the Set-NetIPAddress to enable DHCP for a set of computers so that they would migrate from static IPs to DHCP without needing to touch each individually. This allowed me to migrate an entire floor (about 100 computers) in one night without stepping into the building. That’s the power of automation!
I humbly suggest Test-NetConnection (alias “tnc”) for your next Powershell post.
TNC is the Powershell equivalent of tcping.
It first tries to ping (and you can’t tell it not to), but then will send a SYN to the Port specified, and give you a True/False based on if the ACK comes back.
OK, so it’s more like tcping’s paint-licking cousin. It still gives you some idea of the TCP reachability of a host!
I’ll have to take a look at that. Thanks for the tip.